一、关于Canvas
1. 什么是canvas
1
| <canvas>是一个可以使用脚本(通常为JavaScript)在其中绘制图形的 HTML 元素。
|
2. 基础结构
1 2 3 4 5 6 7 8
| <canvas id="mycanvas" width="200" height="300"> 您的浏览器不支持canvas </canvas>
<script> var cc=document.getElementById("mycanvas"); var cxt=cc.getContext("2d"); </script>
|
canvas 标签只有两个属性—— width和height,这两个属性是可选的。当我们没有定义时,Canvas 的默认大小为300像素×150像素(宽×高,像素的单位是px)。
可以像给其他DOM元素一样给canvas添加样式;当开始时没有为canvas规定样式规则,其将会完全透明。
canvas是一个二维网格,以左上角坐标为(0,0)
3. 绘制矩形
- 参数:
- x与y指定了在canvas画布上所绘制的矩形的左上角(相对于原点)的坐标。
- width和height设置矩形的尺寸。
1
| fillRect( x ,y ,width, height)
|
1
| strokeRect( x ,y ,width, height)
|
4. 绘制路径
图形的基本元素是路径。路径是通过不同颜色和宽度的线段或曲线相连形成的不同形状的点的集合。一个路径,甚至一个子路径,都是闭合的。使用路径绘制图形需要一些额外的步骤。
- 需要创建路径起始点。
- 使用画图命令去画出路径。
- 把路径封闭。
- 一旦路径生成,就能通过描边或填充路径区域来渲染图形。
以下是所要用到的函数:
- 新建一条路径,生成之后,图形绘制命令被指向到路径上生成路径。
这个方法会通过绘制一条从当前点到开始点的直线来闭合图形。如果图形是已经闭合了的,即当前点为开始点,该函数什么也不做。
例子:
1 2 3 4 5 6
| cxt.beginPath(); cxt.moveTo(150,150); cxt.lineTo(150,250); cxt.lineTo(300,250); cxt.stroke(); cxt.closePath();
|
5. 弧线
绘制圆弧或者圆,我们使用arc()方法
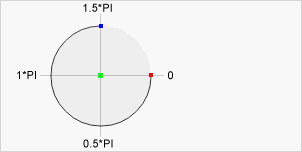
1
| arc(x, y, radius, startAngle, endAngle, anticlockwise)
|
画一个以(x,y)为圆心的以radius为半径的圆弧(圆),从startAngle开始到endAngle结束,参数anticlockwise 为一个布尔值。为true时,是逆时针方向,否则顺时针方向。
注意:arc()函数中的角度单位是弧度,不是度数。角度与弧度的js表达式:radians=(Math.PI/180)*degrees。
例子:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| cxt.beginPath(); cxt.arc(70,70,50,0,Math.PI/2,true); cxt.stroke();
cxt.beginPath(); cxt.arc(180,70,50,0,Math.PI/2,false); cxt.stroke();
cxt.beginPath(); cxt.arc(300,70,50,0,Math.PI/2,true); cxt.fill();
cxt.beginPath(); cxt.arc(400,70,50,0,Math.PI/2,false); cxt.fill();
|
6. 色彩样式
canvas提供了两个色彩属性
注意: 一旦您设置了 strokeStyle 或者 fillStyle 的值,那么这个新值就会成为新绘制的图形的默认值。如果你要给每个图形上不同的颜色,你需要重新设置 fillStyle 或 strokeStyle 的值。
7. 线型的样式
8. 渐变Gradients
我们还可以用线性或者径向的渐变来填充或描边
createLinearGradient(x1, y1, x2, y2) createLinearGradient 方法接受 4 个参数,表示渐变的起点 (x1,y1) 与终点 (x2,y2)。
1 2 3 4 5 6
| var linearGradient=cxt.createLinearGradient(50,50,250,250); linearGradient.addColorStop(0,'yellow'); linearGradient.addColorStop(.5,'red'); linearGradient.addColorStop(1,'green'); cxt.fillStyle=linearGradient; cxt.fillRect(50,50,200,200);
|
9. 绘制文本
在指定的(x,y)位置填充指定的文本,绘制的最大宽度是可选的.
1
| fillText(text, x, y [, maxWidth])
|
1
| strokeText(text, x, y [, maxWidth])
|
二、关于JavaScript
1. setInterval
setInterval
是 JavaScript 中的一个定时器函数,用于周期性地执行指定的代码。
基本语法结构
1 2
| setInterval(function, milliseconds);
|
function
: 要执行的函数或代码块
milliseconds
: 执行间隔时间(毫秒)
使用示例
1 2 3 4 5
| javascript复制代码 setInterval(function() { const date = new Date(); console.log(date.toLocaleTimeString()); }, 1000);
|
注意事项
setInterval
返回一个唯一的标识符,可通过 clearInterval
来清除定时器。
2. Date 对象
- JavaScript 中用于处理日期和时间的内置对象。
- 可以获取当前日期时间,创建特定日期、时间对象。
获取当前日期时间
1 2
| const currentDate = new Date(); console.log(currentDate);
|
获取小时、分钟、秒、毫秒:getHours()
, getMinutes()
, getSeconds()
,getMilliseconds()
三.源码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> </head> <body> <canvas width="500" height="500" id="canvas"></canvas> <script> var canvas = document.getElementById("canvas") var huabi = canvas.getContext("2d")
huabi.strokeStyle = "#0ff"; huabi.lineWidth = 17; huabi.shadowBlur = 15 huabi.shadowColor = "#0ff"
function fun(){
var date = new Date() var today = date.toDateString() var h = date.getHours() var m = date.getMinutes() var s = date.getSeconds() var ms = date.getMilliseconds()
var s1 = s + ( ms / 1000 ); var m1 = m + ( s1 / 60 ) console.log( s1 )
var radialGradient = huabi.createRadialGradient(250,250,20,250,250,300) radialGradient.addColorStop(0,"#03303a") radialGradient.addColorStop(1,"#000") huabi.fillStyle = radialGradient; huabi.fillRect(0,0,500,500)
huabi.beginPath() huabi.arc(250,250,200,270 * (Math.PI / 180),((360 / 12 * h) - 90) * (Math.PI / 180)) huabi.stroke()
huabi.beginPath() huabi.arc(250,250,170,270 * (Math.PI / 180),((360 / 60 * m1) - 90) * (Math.PI / 180)) huabi.stroke()
huabi.beginPath() huabi.arc(250,250,140,270 * (Math.PI / 180),((360 / 60 * s1) - 90) * (Math.PI / 180)) huabi.stroke()
huabi.font = "24px 宋体" huabi.fillStyle = "#0ff" huabi.fillText(today,160,250) }
fun()
setInterval(fun,10) </script> </body> </html>
|